Introduction
In this article, we will explain how to automatically add nodes to Femap using VBA in Excel, which will reduce manual work and make node creation more efficient by utilizing coordinate data written in Excel.
Node creation code
FEMAP provides an API, which can be called in VBA to perform various operations.
Here is an example of VBA code that creates a new node in FEMAP.
The code should be executed after FEMAP has been started.
Sub create_node()
'Create a Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Create a node object
Dim nd As Object
Set nd = f.feNode
'Specify the coordinates of the node
nd.X = 10
nd.Y = 15
nd.Z = 20
nd.put(1) 'Add or overwrite the node at the specified ID.In this case, the node is created with ID number 1.
End Sub
- Lines 4-5 create the Femap object; note that executing this code when FEMAP is not running will result in an error.
- Lines 8-9 create a node object for the Entity object.
- Lines 12-14 set the coordinates of the node to be created. The coordinates are entered in Double type.
- Line 16 nd.put(ID) overwrites the data with the number ID. The ID in parentheses is filled in with the Long type.
Example of linking with Excel sheet
To create a node with values entered in an Excel sheet
Prepare the following sheet in Excel.
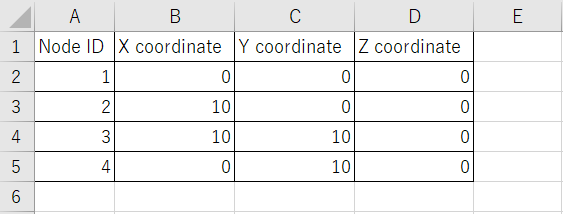
The code to create a node with each value entered on the above sheet is as follows
Sub create_node()
Dim i as Integer
'Create a Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Create a node object
Dim nd As Object
Set nd = f.feNode
For i = 2 To 5
nd.X = Cells(i, 2)
nd.Y = Cells(i, 3)
nd.Z = Cells(i, 4)
nd.Put(Cells(i, 1)) 'Add or overwrite the node data at the specified ID.
Next i
MsgBox "Node creation is complete!”
End Sub
If you get an error when retrieving a value from a cell, you can enclose the part of the value to be retrieved in the cell with CLng() for Long type, CDbl() for Double type, and so on.
Example of exporting node coordinates to Excel
You can also export FEMAP nodes to Excel. In this case, use the .get method.
First, prepare an Excel sheet as shown below. It is almost the same as the aforementioned example.
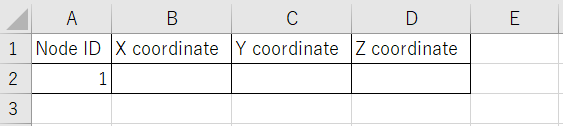
Enter only the ID of the node information you wish to retrieve in the above sheet.
The VBA code is as follows
Sub get_node()
'Create a Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Create a node object
Dim nd As Object
Set nd = f.feNode
nd.Get(Int(Cells(2,1))) 'Retrieve the node data for the specified ID
Cells(2,2) = nd.X 'Get the X coordinate
Cells(2,3) = nd.Y 'Get the Y coordinate
Cells(2,4) = nd.Z 'Get the Z coordinate
End Sub
- Line 11 nd.get(ID) obtains the data of the number ID. The ID in parentheses is filled in with the Long type. When obtaining cell values, you can reduce the possibility of errors by converting the type, such as CLng(Cells(2,1)).
- If you run this code, you can have the node information output to the Excel sheet.
Conclusion
With the above procedure, you can create nodes in Femap utilizing VBA in Excel. By using this method, you can easily import coordinate data into Femap’s model while managing them in Excel. Please try this method because it is very useful to improve efficiency of design and to prevent input errors.
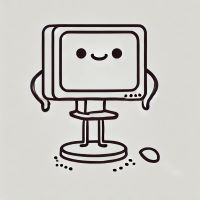
Nodes are the foundation. Without them, your analysis will be completely lost—like a GPS without satellites!
コメント