Introduction.
Managing the locking/unlocking of layers is very important to improve your work efficiency in AutoCAD.
This article details how to lock and unlock layers in AutoCAD using Excel VBA.
Lock/Unlock settings for layers
Basic VBA Code
First, here is some basic VBA code to lock and unlock a picture layer in AutoCAD.
This code switches the picture layer to locked when it is unlocked and unlocks it when it is locked.
Sub ToggleLayerLock()
Dim acadApp As Object
Dim acadDoc As Object
Dim layer As Object
'Get the AutoCAD application
Set acadApp = getObject(,"AutoCAD.Application")
Set acadDoc = acadApp.ActiveDocument
'Get the target layer
Set layer = acadDoc.Layers.Item("LayerToLock")
'Toggle layer lock/unlock
If layer.Lock Then
layer.Lock = False
Else
layer.Lock = True
End If
End Sub
Detailed Description
Get the AutoCAD application using the GetObject function.
Get the current document using the ActiveDocument property.
Use the Layers.Item method to retrieve the target image layer.
Use the Lock property to toggle locking and unlocking of the layers.
Layer lock/unlock application
Locking a specific layer
First, here is the basic VBA code to lock the layer in AutoCAD.
Sub LockLayer()
Dim acadApp As Object
Dim acadDoc As Object
Dim layer As Object
'Get the AutoCAD application
Set acadApp = getObject(,"AutoCAD.Application")
Set acadDoc = acadApp.ActiveDocument
'Get the target layer
Set layer = acadDoc.Layers.Item("LayerToLock")
'Lock the layer
layer.Lock = True
End Sub
Detailed Description
Same as described earlier, but the picture layer can be locked by setting the Lock property to True.
Unlock specific picture layers
Basic VBA Code
Next, here is the basic VBA code to unlock the picture layer in AutoCAD.
Sub UnlockLayer()
Dim acadApp As Object
Dim acadDoc As Object
Dim layer As Object
'Get the AutoCAD application
Set acadApp = getObject(,"AutoCAD.Application")
Set acadDoc = acadApp.ActiveDocument
'Get the target layer
Set layer = acadDoc.Layers.Item("LayerToUnlock")
'Unlock the layer
layer.Lock = False
End Sub
Detailed Description
Same as described earlier, but the picture layer can be unlocked by setting the Lock property to False.
summary
Managing the locking/unlocking of picture layers is a fundamental step in streamlining your work with AutoCAD, and VBA allows you to automate these operations and save time.
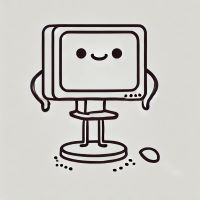
I locked the layer. Now even Thanos can’t erase my lines.
コメント