Introduction
In this article, we will show you how to draw ellipses in AutoCAD using Excel VBA. Ellipses may not seem to be used very often, but they are used for pipe cross-sections, windows of buildings with designs, and other unexpected opportunities. Of course, it is possible to link this with Excel and have it automatically drawn. Let’s try it.
Sample code for drawing an ellipse
Let me introduce you to a sample code that draws an ellipse using the values entered into Excel. First, prepare the following Excel sheet.

Cells A2 to D2 are input cells, and E2 is a cell to retrieve and store handle values after drawing, so it can be left blank when drawing.
Sub DrawEllipseInAutocad()
'Declare the AutoCAD application
Dim acadApp As Object
Dim acadDoc As Object
'Start or retrieve AutoCAD
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("Autocad.application")
End If
On Error GoTo 0
'Create or retrieve an AutoCAD document
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'Prepare variables
Dim centerX As Double 'Variable to store the X-coordinate of the ellipse's center
Dim centerY As Double 'Variable to store the Y-coordinate of the ellipse's center
Dim xAxisRadius As Double 'Variable to store the X-axis radius of the ellipse
Dim yAxisRadius As Double 'Variable to store the Y-axis radius of the ellipse
'Retrieve values from the Excel sheet
centerX = Cells(2, 1)
centerY = Cells(2, 2)
xAxisRadius = Cells(2, 3)
yAxisRadius = Cells(2, 4)
'Error check
If xAxisRadius <= 0 Or yAxisRadius <= 0 Then
MsgBox "The radii of the X-axis and Y-axis must be positive values.", vbCritical
Exit Sub
End If
'Define the center point and radii of the ellipse
Dim center(0 To 2) As Double 'Array to store the center coordinates
Dim majorAxis(0 To 2) As Double 'Array to store the end point of the major axis
Dim ratio As Double 'Ratio of the major axis to the minor axis; must be 1 or less
'Input the center of the ellipse
center(0) = centerX
center(1) = centerY
center(2) = 0 'Z-coordinate; set to 0 to draw on the XY plane
'Determine whether the ellipse is wider or taller, and assign the major and minor axes accordingly
If yAxisRadius > xAxisRadius Then
'Set the major axis in the Y direction
majorAxis(0) = 0
majorAxis(1) = yAxisRadius
majorAxis(2) = 0
ratio = xAxisRadius / yAxisRadius
Else
'Set the major axis in the X direction
majorAxis(0) = xAxisRadius
majorAxis(1) = 0
majorAxis(2) = 0
ratio = yAxisRadius / xAxisRadius
End If
'Create the ellipse
Dim ellipseObj As Object
Set ellipseObj = acadDoc.modelSpace.AddEllipse(center, majorAxis, ratio)
'Retrieve the handle
Cells(2,5) = ellipseObj.Handle
'Display AutoCAD
acadApp.Visible = True
'Show a message
MsgBox ”The ellipse has been drawn!”
End Sub
VBA Funtion procedure to draw an ellipse (Ellipse)
If you create your code as a Function procedure, you can more easily draw ellipses. Here we describe how to do it.
VBA Preparation
The method I am about to show you requires you to set references to the AutoCAD object library in order to manipulate AutoCAD with Excel VBA.
From the VBA editor menu, select “Tools” -> “Reference Settings”.
Select “AutoCAD Type Library” or “AutoCAD Object Library” from the list, check the box and click “OK”.
(*The name may change slightly depending on the version. AutoCAD 2021 Type Library” etc.)
Sample Function Procedure Code
First, copy and paste the code below in the EXCEL VBA standard module as is.
You can copy it with one click by pressing the button in the upper right corner of the code window below.
Public acadApp As AcadApplication 'Variable to hold the AutoCAD application object
Public acadDoc As AutoCAD.AcadDocument 'Variable to hold the AutoCAD document object
Function DrawEllipseInAutoCAD(centerX As Double, centerY As Double, xAxisRadius As Double, yAxisRadius As Double) As String
'Function to draw an ellipse in AutoCAD
'The arguments are, in order:
'X-coordinate of the center, Y-coordinate of the center, X-axis radius, Y-axis radius
'The return value is the handle of the drawn ellipse
Dim ellipseObj As AcadEllipse 'Variable to hold the ellipse object
'Define the center point and radii of the ellipse
Dim center(0 To 2) As Double 'Array to store the center coordinates
Dim majorAxis(0 To 2) As Double 'Array to store the end point of the major axis
Dim ratio As Double 'Ratio of the major axis to the minor axis; must be 1 or less
'Input the center of the ellipse
center(0) = centerX
center(1) = centerY
center(2) = 0 'Z-coordinate; set to 0 to draw on the XY plane
'Determine whether the ellipse is wider or taller, and assign the major and minor axes accordingly
If yAxisRadius > xAxisRadius Then
'Set the major axis in the Y direction
majorAxis(0) = 0
majorAxis(1) = yAxisRadius
majorAxis(2) = 0
ratio = xAxisRadius / yAxisRadius
Else
'Set the major axis in the X direction
majorAxis(0) = xAxisRadius
majorAxis(1) = 0
majorAxis(2) = 0
ratio = yAxisRadius / xAxisRadius
End If
'Add the ellipse to the model space in AutoCAD
Set ellipseObj = acadDoc.ModelSpace.AddEllipse(center, majorAxis, ratio)
'Return the handle of the added ellipse
DrawEllipseInAutoCAD = ellipseObj.Handle
End Function
Now drawing an ellipse is as simple as calling DrawEllipseInAutoCAD(centerX, centerY , xAxisRadius, yAxisRadius).
For example, prepare the following sheet
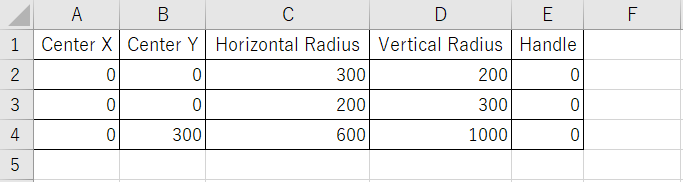
Then write a sample of the main procedure.
public Sub main()
On Error GoTo OUT1 'Error handling: When AutoCAD is not running
Set acadApp =GetObject(,"AutoCAD.Application") 'Retrieve the existing AutoCAD application
On Error GoTo OUT2 'Error handling: When no file is open in AutoCAD
Set acadDoc = acadApp.ActiveDocument 'Retrieve the active AutoCAD document
On Error GoTo 0 'Reset error handling
'Draw ellipses in AutoCAD and write their handles to Excel
Cells(2, 5) = DrawEllipseInAutoCAD(Cells(2, 1), Cells(2, 2), Cells(2, 3), Cells(2, 4))
Cells(3, 5) = DrawEllipseInAutoCAD(Cells(3, 1), Cells(3, 2), Cells(3, 3), Cells(3, 4))
Cells(4, 5) = DrawEllipseInAutoCAD(Cells(4, 1), Cells(4, 2), Cells(4, 3), Cells(4, 4))
Exit Sub
OUT1:
MsgBox "Please start AutoCAD"
Exit Sub
OUT2:
MsgBox "Please open a file in AutoCAD"
End Sub
Lines 11 to 13 are calling the Function procedure. For the sake of the sample, I am describing each one individually, but you can draw a large number of ellipses by using a For statement, etc.
Of course, you can use the following without reading values from cells.
Cells(2, 5) = DrawEllipseInAutoCAD(500, 500, 600, 400) 'Draws an ellipse with the center at (500, 500), a vertical radius of 600, and a horizontal radius of 400
If you want to draw an ellipse but do not need a handle, you can prepare a variable such as dummy as shown below.
dummy = DrawEllipseInAutoCAD(500, 500, 600, 400) 'Draws an ellipse with the center at (500, 500), a vertical radius of 600, and a horizontal radius of 400
Conclusion
In this article, we introduced a standard code for automatically drawing ellipses using Excel and a Function procedure for added convenience. By applying this method and combining it with other drafting methods, you can draw complex shapes in AutoCAD based on data entered in Excel or achieve complete automatic drafting. Please try to get more complex operations little by little.
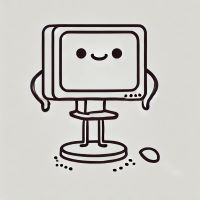
Ellipses? It’s a stretched version of a circle.
コメント