Introduction.
Hello, I have a question! In this article, I will show you how to draw polylines in AutoCAD using Excel VBA.
AutpCAD is a powerful design tool, but it can be automated and made more efficient by using VBA.
I will explain it in a way that is easy to understand even for beginners, so please stay with me until the end.
VBA Preparation
First, prepare to use VBA.
Open the editor for VBA in Excel
Open Excel and press “Alt + F11” to launch the VBA editor.
Perform reference setting
In the VBA editor, select “Tools” > “Reference Settings” and check “AutoCAD Type Library”.
This will allow you to manipulate AutoCAD objects from VBA.
(However, the reference setting may not be necessary for this article.)
Sample code for drawing a polyline
Below is a sample code for drawing a polyline.
Sub CreatePolylineInAutoCAD()
Dim acadApp As Object
Dim acadDoc As Object
Dim polyline As Object
Dim pointNum As Long 'Number of vertices for the polyline
Dim points() As Double 'Array to store the coordinates of the polyline vertices
Dim handle As String 'Variable to store the handle of the polyline
'Launch AutoCAD or get the running instance
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("AutoCAD.Application")
End If
On Error GoTo 0
'Make AutoCAD visible
acadApp.Visible = True
'Create a new AutoCAD document or get the active one
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'Define the number of vertices for the polyline
pointNum = 5
ReDim points((pointNum -1) * 2 + 1) 'Resize the points array to match the number of vertices
'Set the coordinates of the polyline vertices (even indices are X-coordinates, odd indices are Y-coordinates)
points(0) = 0 : points(1) = 5
points(2) = -3 : points(3) = -4
points(4) = 4.75 : points(5) = 1.5
points(6) = -4.75 : points(7) = 1.5
points(8) = 3 : points(9) = -4
'Create the polyline
Set polyline = acadDoc.ModelSpace.AddLightWeightPolyline(points)
'Close the polyline (True to close it, False to leave it open)
polyline.Closed = True
'Get the handle of the polyline
handle = polyline.Handle
'Show a message box with the handle information
MsgBox "The polyline has been created. Handle of the polyline: " & handle
'Make AutoCAD visible
acadApp.Visible = True
End Sub
- Lines 10 to 16 retrieve AutoCAD if it is running, or start a new one if it is not running.
- Lines 21-27 retrieve the file if it is open in AutoCAD, or open a new file if not.
- Lines 30-31 create an array to store the polyline vertices. An array is needed to specify the vertices of the polyline. The size of the array must be even.
- The coordinates of the vertices are entered in lines 33-38. In order, we store the X coordinate of point 1, the Y coordinate of point 1, the X coordinate of point 2, the Y coordinate of point 2, and so on.
- Line 41, acadDoc.ModelSpace.AddLightWeightPolyline(points), is the code that creates the polyline. The argument is an array of coordinates of the vertices we just createdす。
Editing Notes
When editing the above polyline creation code, please note the following.
Put the number of vertices of the polyline in pointNum. This is used to change the size of the points() array.
Adjust the number and content of points() according to the number and position of the vertices. points() stores the X and Y coordinates of the first point, the X and Y coordinates of the second point, the X and Y coordinates of the third point, and so on, in that order.
Conclusion
How was it? In this article, we have shown you how to draw polylines in AutoCAD using Excel VBA.
By using VBA, you can improve the efficiency of your design work, so please give it a try.
I hope this article will be useful to you. I hope you enjoy using VBA and AutoCAD!
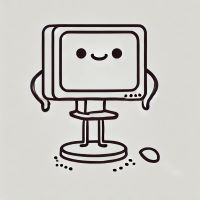
“My polyline automation script isn’t working…”
“Looks like you forgot to ‘close’ the loop!”
コメント