Introduction
Managing layers is very important to improve the efficiency of your work in AutoCAD.
This article explains how to create, delete and activate layers in AutoCAD using EXCEL VBA.
Basic VBA Code
layer activation
First, here is the VBA code to activate an existing layer.
Sub ActiveLayer()
Dim acadApp As Object
Dim acadDoc As Object
Dim layer As Object
'Get the AutoCAD application
Set acadApp = GetObject(,"AutoCAD.Application")
Set acadDoc = acadApp.ActiveDocument
'Retrieve the layer by its name
On Error Resume Next
Set layer = acadDoc.Layers.Item("LayerName")
On Error GoTo 0
'Handle the case where the specified layer does not exist
If layer Is Nothing Then
MsgBox "The specified layer does not exist."
Exit Sub
End If
'Set the layer as the active layer
acadDoc.ActiveLayer = layer
End Sub
Brief Description
The layer can be activated by setting ThisDrawing.ActiveLayer.
Create a new picture layer
Next, we will show you the basic VBA code to create a new picture layer in AutoCAD.
Sub CreateLayer()
Dim acadApp As Object
Dim acadDoc As Object
Dim newLayer As Object
'Get the AutoCAD application
Set acadApp = GetObject(,"AutoCAD.Application")
Set acadDoc = acadApp.ActiveDocument
'Create a new layer
Set newLayer = acadDoc.Layers.Add("NewLayerName")
'Set properties for the new layer
newLayer.Color = acRed
newLayer.LineType = "Continuous"
End Sub
Detailed Description
Get the AutoCAD application using the GetObject function.
Get the current document using the ActiveDocument property.
Add method to create a new layer and set its name.
Set the layer attributes using the Color and LineType properties.
Deletion of a layer
Finally, here is the basic VBA code to delete an existing layer.
Sub DeleteLayer()
Dim acadApp As Object
Dim acadDoc As Object
Dim delLayer As Object
'Get the AutoCAD application
Set acadApp = GetObject(,"AutoCAD.Application")
Set acadDoc = acadApp.ActiveDocument
'Retrieve the layer to be deleted
On Error Resume Next
Set delLayer = acadDoc.Layers.Item("NameOfLayerToDelete")
On Error GoTo OUT1
'Check if the specified layer exists
If delLayer Is Nothing Then
MsgBox "The specified layer does not exist."
Exit Sub
End If
'Delete the specified layer
delLayer.Delete
MsgBox "The specified layer has been deleted successfully."
Exit Sub
OUT1:
MsgBox "An error occurred: " & Err.Description
End Sub
Detailed Description
Use the Layers.item method to retrieve the layers to be deleted.
Use the delete method to delete a layer.
conclusion
Creating and deleting picture layers are fundamental steps in streamlining your work with AutoCAD, and VBA can help you automate these operations and make your time easier to preach.
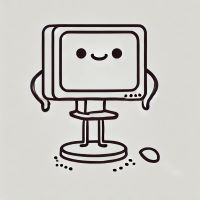
Why did the AutoCAD user bring a flashlight to work?
Because they got lost in all the layers!
コメント