Introduction
Femap is a powerful tool for finite element analysis (FEA), but it is time-consuming when there are many geometry and property settings. In such a case, automation using EXCEL VBA is useful. This article explains how to create a property of “T-Shaped Section Beam” in Femap. It will be explained with codes, so beginners don’t need to worry!
Beam creation code for property T-shaped cross section
First, make sure that the material has already been created before creating the beam. The following related article also explains how to create a material using Excel.
Now, here is an example of VBA code that creates a new T-shaped beam in FEMAP.
The code must be run after FEMAP has been started.
Sub CreatTBeam
'Create a Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Create a property object
Dim pr As Object
Set pr = f.feProp
'
Dim rc As Long
'Input the dimensions for the T-beam cross-section
Dim shape(5) As Double
shape(0) = 134 'Enter the height. In this case, the height will be 150.
shape(1) = 75 'Enter the top flange width. In this case, the width will be 75.
shape(2) = 0 'T型ビームの場合、ここには0を入力。
shape(3) = 16 'Enter the thickness of the top flange. In this case, the thickness will be 16.
shape(4) = 0 'For a T-beam, set this to 0.
shape(5) = 12 'Enter the thickness of the vertical web. In this case, the thickness will be 12.
'Set the orientation direction: 0: Right, 1: Up, 2: Left, 3: Down
Dim orient As Long
orient = 0
pr.title = "150X75X12X16 T" 'タSet the title. In this case, "150X75X12X16 T".
pr.matlID = 1 'Set the material ID. In this case, 1.
pr.Type = 5 'Set the property type. For beams, use 5.
'Create the property
rc = pr.ComputeStdShape(12, shape, orient, 0, True, True, False) 'Parameters: Shape ID, cross-section definition parameters, orientation, evaluation method, shear center offset calculation, torsional constant calculation, stress recovery points
pr.Put(1) 'Register the created property with Property ID 1.
'Release objects
Set pr = Nothing
Set f = Nothing
if rc = 0 then
MsgBox "Failed to create property."
else
MsgBox "Property created successfully."
End If
End Sub
- Lines 4-5 create the Femap object; note that executing this code when FEMAP is not running will result in an error.
- Lines 8-9 create the property object of the Entity object.
- Line 12 is the creation of the variable used to receive the return code, which should be of type Long.
- Lines 15-21 are the input of the shape, which should be of type Double; for T-shaped steel, the key is to assign 0 to shape(2) and shape(4).
- Lines 24-25 are the orientation direction inputs, which are of type Long, and should be entered as integers from 0 to 3.
- The direction of orientation is 0: right, 1: up, 2: left, 3: down, and so on.
- Line 27 assigns the title, using the String type.
- Line 28 assigns the material ID, which is of type Long.
- Line 29, “.Type” is used to input the type. In this case, we are creating a beam, so we specify “5”.
- “ComputeStdShape()” on line 32 creates the property.
- The seven arguments are, from left to right: shape ID (set to 12 this time because it is a T-shaped cross section), cross section definition parameter array, orientation direction, cross section evaluation method, shear center offset variable, torsion constant calculation, and stress extraction position.
- The cross-section evaluation method is specified by a Long type: 0: Automatic, 1: Original, 2: Alternative cross-section performance, and 3: Nastran PBEAML.
- Shear center offset is specified by Boolean; if True, shear center offset is calculated.
- Torsion constant calculation is a Boolean; if True, torsion constants are calculated.
- The stress takeoff location is also specified as a Boolean; if True, the calculation will use the stress takeoff location and orientation currently defined for the object; if False, the stress takeoff location and orientation will be the default.
- Line 33, “.Put()” registers the generated property with the specified property ID.
- Lines 36-37 are object release. There is no need to do so.
- Lines 39-43, if the property is not created, 0 is returned in the return code.
Example of linking to an Excel sheet
To create the properties of a T-shaped section beam with the values entered in an Excel sheet
Prepare the following sheet in Excel.
The input columns are from A2 to H2.

The code to create a property with each value entered on the above sheet is as follows
Sub CreatTBeam
'Create a Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Create a property object
Dim pr As Object
Set pr = f.feProp
'Variable to store the return code
Dim rc As Long
'Input the dimensions for the T-beam cross-section
Dim shape(5) As Double
shape(0) = Cells(2, 4) 'Enter the height.
shape(1) = Cells(2, 5) 'Enter the top flange width.
shape(2) = 0 'For a T-beam, set this to 0.
shape(3) = Cells(2, 6) 'Enter the thickness of the top flange.
shape(4) = 0 'For a T-beam, set this to 0.
shape(5) = Cells(2, 7) 'Enter the thickness of the vertical web.
'et the orientation direction: 0: Right, 1: Up, 2: Left, 3: Down
Dim orient As Long
orient = Cells(2, 8)
pr.title = Cells(2,3) 'Set the title.
pr.matlID = Cells(2,2) 'Set the material ID.
pr.Type = 5 'Set the property type. For beams, use 5.
'Create the property
rc = pr.ComputeStdShape(12, shape, orient, 0, True, True, False) 'Parameters: Shape ID, cross-section definition parameters, orientation, evaluation method, shear center offset calculation, torsional constant calculation, stress recovery points
pr.Put(Cells(2,1)) 'Register the created property with the specified Property ID.
'Release objects
Set pr = Nothing
Set f = Nothing
if rc = 0 then
MsgBox "Failed to create property."
else
MsgBox "Property created successfully."
End If
End Sub
Example of exporting property information to Excel
You can also export the property information of Femap to Excel, prepare the following table as you did for I-beam and C-beam.
First, prepare an Excel sheet as shown below.
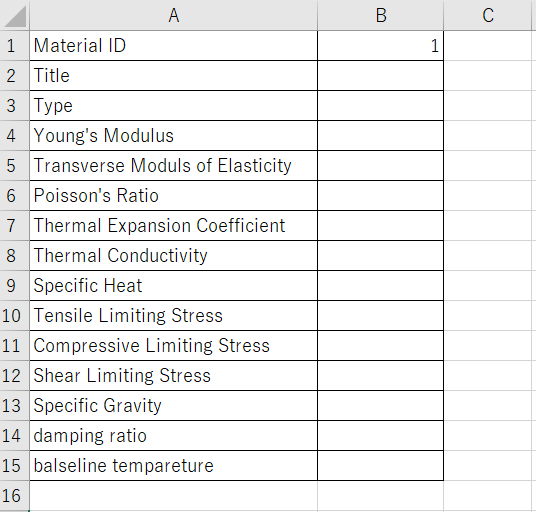
Enter only the number of the property ID you want to retrieve in the sheet above.
The VBA code will look like this
By executing this code, you can retrieve the property information in each blank field.
Sub GetBeamProperty()
On Error GoTo OUT1
'Create a FEMAP model object
Dim f As Object
Set f = GetObject(,"femap.model")
On Error GoTo OUT2
'Create a property object
Dim pr As Object
Set pr = f.feProp
Dim rc As Long 'Return code
pr.Get(Int(Cells(1,2))) 'Retrieve the property ID specified in cell B1
Cells(2,2) = pr.matlID 'Retrieve the material ID
Cells(3,2) = pr.title 'Retrieve the title
Cells(4,2) = pr.Type 'Retrieve the type. For beam elements, this will be 5.
'If the retrieved property is a beam, retrieve additional parameters
If pr.Type = 5 Then
Cells(5,2) = pr.pval(40) 'General section height
Cells(6,2) = pr.pval(41) 'General section top flange width
Cells(7,2) = pr.pval(42) 'General section bottom flange width
Cells(8,2) = pr.pval(43) 'General section top flange thickness
Cells(9,2) = pr.pval(44) 'General section bottom flange thickness
Cells(10,2) = pr.pval(45) 'General section web thickness
Cells(11,2) = pr.pval(0) 'Cross-sectional area at endpoint A
Cells(12,2) = pr.pval(5) 'Effective Y-sectional area at endpoint A
Cells(13,2) = pr.pval(6) 'Effective Z-sectional area at endpoint A
Cells(14,2) = pr.pval(1) 'I1 at endpoint A
Cells(15,2) = pr.pval(2) 'I2 at endpoint A
Cells(16,2) = pr.pval(16) 'Neutral axis offset Y at endpoint A
Cells(17,2) = pr.pval(17) ' Neutral axis offset Z at endpoint A
Else
MsgBox "The property " & Cells(1,2) & " is not a beam."
End If
'Release objects
Set pr = Nothing
Set f = Nothing
Exit Sub
OUT1:
MsgBox "Please start FEMAP."
Exit Sub
OUT2:
MsgBox "Unknown error."
End Sub
- The property is obtained by “.get(property ID)” on line 15. It is better to specify the type with Int() to prevent errors. (The same is true for other cases of taking values from cells.)
- Various information is obtained by “.pval(number)” in lines 23-35. The number is determined by the information to be obtained, and in the case of a beam, it is as commented above.
- However, in the case of a T-type beam, there is no plate that corresponds to the underside of an I-type beam. The part corresponding to the lower plate width and thickness will be 0.
Conclusion
This article explains how to create T-type beam properties in Femap using EXCEL VBA. By automating the process, you can greatly reduce the time and effort to set up and prevent mistakes. Please try to apply it to your own analysis!
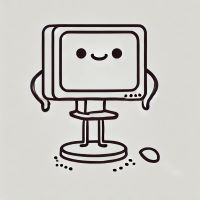
Designing T-sections: because sometimes you just need a strong T(tea).
コメント