はじめに
Hello, I’m looking for a way to create a property for an I-beam in Femap using Excel VBA! In this article, we will discuss how to automatically create I-beam properties in Femap using Excel VBA.Femap is a powerful tool for utilizing the Finite Element Analysis (FEA), but manually entering beam properties can be time consuming. So, use Excel VBA to improve efficiency.
Property I Beam Creation Code
FEMAP also provides an API for creating properties. This can be used to create a variety of properties.
First, a material must be created before the beam can be created. Please refer to the following related article on how to create materials using Excel.
Here is an example of VBA code that creates a new I-beam in FEMAP.
The code must be executed after FEMAP has been started.
Sub CreatIBeam
'Creating a Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Creating Property Objects
Dim pr As Object
Set pr = f.feProp
'Where return codes are stored
Dim rc As Long
'Input of geometry(For I-shaped cross section)
Dim shape(5) As Double
shape(0) = 400 'Input the height. In this case, the height is 400.
shape(1) = 400 'Top flange width. In this case, the width is 400.
shape(2) = 400 'Bottom flange width. In this case, the width is 400.
shape(3) = 16 'Top flange thickness. In this case, it is 16.
shape(4) = 16 'Bottom flange thickness. In this case, it is 16.
shape(5) = 12 'Web thickness. In this case, it is 12.
'Set the orientation direction. 0: right, 1: up, 2: left, 3: down
Dim orient As Long
orient = 0
pr.title = "400X400X12/16 I" 'Input title. In this case, “400X400X12/16 I”.
pr.matlID = 1 'Enter the material ID. In this case, it is 1.
pr.Type = 5 'Enter the type. For beams, use 5.
'Creating the property
rc = pr.ComputeStdShape(9, shape, orient, 0, True, True, False) 'Arguments: shape ID, cross-section definition parameters, orientation, cross-section evaluation method, shear center offset calculation, torsional constant calculation, stress extraction location
pr.Put(1) 'Register the created property with property ID 1.
'Releasing objects
Set pr = Nothing
Set f = Nothing
if rc = 0 then
MsgBox "Failed to create property"
else
MsgBox "Property created successfully"
End If
End Sub
- Lines 4-5 create the Femap object; note that executing this code when FEMAP is not running will result in an error.
- Lines 8-9 create the property object of the Entity object.
- Line 12 is the creation of the variable used to receive the return code, which should be of type Long.
- Lines 15-21 are the input of the shape, which should be of type Double.
- Lines 24-25 are the orientation direction inputs, of type Long, with integers from 0 to 3.
- The orientation direction is 0: right, 1: up, 2: left, 3: down, and so on.
- Line 27 assigns the title, using the String type.
- Line 28 assigns the material ID, which is of type Long.
- Line 29, “.Type” is used to input the type. In this case, we are creating a beam, so we specify “5”.
- ComputeStdShape()” on line 32 creates the property. The seven arguments are, from left to right: shape ID (set to 9 this time because it is an I-shaped cross-section), cross-section definition parameter array, orientation direction, cross-section evaluation method, shear center offset variable, torsion constant calculation, and stress extraction position.
- The cross-section evaluation method is specified by a Long type: 0: Automatic, 1: Original, 2: Alternative cross-section performance, and 3: Nastran PBEAML.
- Shear center offset is specified by Boolean; if True, shear center offset is calculated.
- Torsion constant calculation is specified by Boolean; if True, torsion constants are calculated.
- The stress takeoff location is also specified by Boolean; if True, the calculation will use the stress takeoff location and orientation currently defined for the object; if False, the stress takeoff location and orientation will be the default.
- Line 33, “.Put()” registers the generated property with the specified property ID.
- Lines 36-37 are object release. There is no problem without it. When creating a large program, it is better to release unneeded objects in this way to make the program lighter.
- Lines 39 to 43 return 0 if the property is not created.
Example of linking to an Excel sheet
To create I-beam properties with values entered on an Excel sheet
Prepare the following sheet in Excel.
The input columns are A2 to J2.

The code to create a property with each value entered on the above sheet is as follows.
Sub CreatIBeam
'Create Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Create property object
Dim pr As Object
Set pr = f.feProp
'Storage for the return code
Dim rc As Long
'Storage for the return code
Dim shape(5) As Double
shape(0) = Cells(2,4) 'Input height
shape(1) = Cells(2,5) 'Top flange width
shape(2) = Cells(2,6) 'Bottom flange width
shape(3) = Cells(2,7) 'Top flange thickness
shape(4) = Cells(2,8) 'Bottom flange thickness
shape(5) = Cells(2,9) 'Web thickness
'Set the orientation direction. 0: right, 1: up, 2: left, 3: down
Dim orient As Long
orient = Cells(2,10)
pr.title = Cells(2,3) 'Input title
pr.matlID = Cells(2,2) 'Input material ID
pr.Type = 5 'Input material ID
'Create the property
rc = pr.ComputeStdShape(9, shape, orient, 0, True, True, False) 'Arguments: shape ID, cross-section definition parameters, orientation, cross-section evaluation method, shear center offset calculation, torsional constant calculation, stress extraction location
pr.Put(Cells(2,1)) 'Register the created property with the specified ID number
'Release objects
Set pr = Nothing
Set f = Nothing
if rc = 0 then
MsgBox "Failed to create property"
else
MsgBox "Property created successfully"
End If
End Sub
Example of exporting property information to Excel
You can also export the property information of Femap to Excel, and you can get the following values for I-type beams.
First, prepare an Excel sheet as shown below.
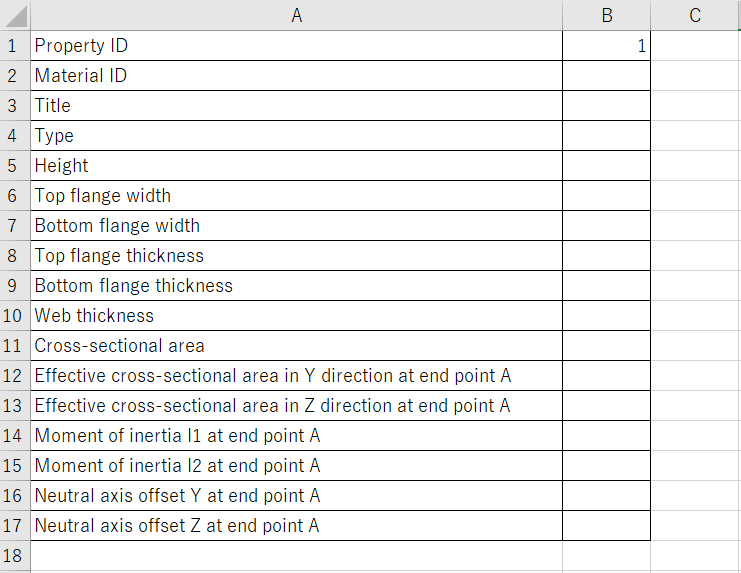
Enter only the number of the property ID you want to retrieve in the sheet above.
The VBA code will look like this
By executing this code, you can retrieve the property information in each blank field.
Sub GetBeamProperty()
On Error GoTo OUT1
'Generate FEMAP model object
Dim f As Object
Set f = GetObject(,"femap.model")
On Error GoTo OUT2
'Generate property object
Dim pr As Object
Set pr = f.feProp
Dim rc As Long 'Return code
pr.Get(Int(Cells(1,2))) 'Retrieve the property ID entered in cell B1
Cells(2,2) = pr.matlID 'Retrieve material ID
Cells(3,2) = pr.title 'Retrieve title
Cells(4,2) = pr.Type 'Retrieve type (should be 5 for beam elements)
'If the retrieved property is a beam, retrieve the following items
If pr.Type = 5 Then
Cells(5,2) = pr.pval(40) 'General section height
Cells(6,2) = pr.pval(41) 'General section top flange width
Cells(7,2) = pr.pval(42) 'General section bottom flange width
Cells(8,2) = pr.pval(43) 'General section top flange thickness
Cells(9,2) = pr.pval(44) 'General section bottom flange thickness
Cells(10,2) = pr.pval(45) 'General section web thickness
Cells(11,2) = pr.pval(0) 'Cross-sectional area at end point A
Cells(12,2) = pr.pval(5) 'Effective cross-sectional area in Y direction at end point A
Cells(13,2) = pr.pval(6) 'Effective cross-sectional area in Z direction at end point A
Cells(14,2) = pr.pval(1) 'Moment of inertia I1 at end point A
Cells(15,2) = pr.pval(2) 'Moment of inertia I2 at end point A
Cells(16,2) = pr.pval(16) 'Neutral axis offset Y at end point A
Cells(17,2) = pr.pval(17) 'Neutral axis offset Z at end point A
Else
MsgBox "This property " & Cells(1,2) & " is not a beam."
End If
'Release objects
Set pr = Nothing
Set f = Nothing
Exit Sub
OUT1:
MsgBox "Please start FEMAP"
Exit Sub
OUT2:
MsgBox "Unknown error"
End Sub
- The property is obtained by “.get(property ID)” on line 15. It is better to specify the type with Int() to prevent errors. (The same is true for other cases of taking values from cells.)
- Various information is obtained by “.pval(number)” in lines 23-35. The number is determined by the information to be obtained, and in the case of a beam, it is as shown in the above comment.
Conclusion
In this article, we described the basic method of creating I-beam properties in Femap using Excel VBA. By automating manual tasks, the efficiency of design work can be greatly improved. Please try to improve your design process by automating other properties and settings via VBA!
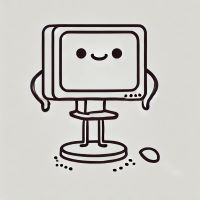
I realized that automating the creation of the I-beam and saving time was saving me (I) time.
コメント