Introduction
The integration of AutoCAD and Excel VBA is one way to greatly improve operational efficiency. This article will show you how to insert text into AutoCAD using Excel VBA.
Preparation of VBA in EXCEL
In order to manipulate AutoCAD with Excel VBA, the AutoCAD object library must be set as a reference.
- Select “Tools” -> “Reference Settings” from the VBA Editor menu.
- Select “AutoCAD Type Library” or “AutoCAD Object Libraly” from the list, check the box and click “OK”.
(*The name may change slightly depending on the version. AutoCAD 2021 Type Library” etc.)
Sample code to insert a string (text)
Let me show you a sample code to insert text using the values entered in Excel.
First, prepare an Excel sheet like the one below.

A2 to G2 are input cells, and H2 is a cell to get the handle of the inserted string. No input is required when drawing. The text reference position is set as follows.
origin position | Excel input character | VBA Code | reference number |
---|---|---|---|
Top Left | TL | acAlignmentTopLeft | 6 |
Top Center | TC | acAlignmentTopCenter | 7 |
Top Right | TR | acAlignmentTopRight | 8 |
Middle Left | ML | acAlignmentMiddleLeft | 9 |
Middle Center | MC | acAlignmentMiddleCenter | 10 |
Middle Right | MR | acAlignmentMiddleRight | 11 |
Left | L | acAlignmentLeft | 0 |
Center | C | acAlignmentCenter | 1 |
Right | R | acAlignmentRight | 2 |
Bottom Left | BL | acAlignmentBottomLeft | 12 |
Bottom Center | BC | acAlignmentBottomCenter | 13 |
Bottom Right | BR | acAlignmentBottomRight | 14 |
VBAコードはつぎのようになります。
Sub AddTextInAutoCAD()
Dim acadApp As Object 'Declare an AutoCAD application object
Dim acadDoc As Object 'Declare an AutoCAD document object
Dim textObj As Object 'Declare character objects
Dim insertPoint(0 To 2) As Double 'character position
Dim text As String 'Content of string to be filled in
Dim textHeight As Double 'graphic size
Dim textRotation As Double 'Character Angles
Dim textAlignment As String 'character base station
'Launch or retrieve AutoCAD
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("AutoCAD.Application")
End If
On Error GoTo 0
'Show AutoCAD
acadApp.Visible = True
'Create or retrieve a new AutoCAD document.
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'Set text insertion position, content, size, and angle
text = Cells(2,1) 'text content
textHeight = Cells(2,2) 'graphic size
textRotation = Cells(2,3) * (3.14159265358979 / 180) 'Character angle. Converted to radians.
textAlignment = Cells(2,4) 'character base station
insertPoint(0) = Cells(2,5) 'X-coordinate
insertPoint(1) = Cells(2,6) 'Y-coordinate
insertPoint(2) = Cells(2,7) 'Z-coordinate
'text generation
Set textObj = acadDoc.modelSpace.AddText(text, insertPoint, textHeight)
'Set text rotation angle
textObj.Rotation = textRotation
'Set character base point
Select Case textAlignment
Case "TL"
textObj.Alignment = acAlignmentTopLeft 'top-let(TL)
Case "TC"
textObj.Alignment = acAlignmentTopCenter 'top-center(TC)
Case "TR"
textObj.Alignment = acAlignmentTopRight 'tiop-right(TR)
Case "ML"
textObj.Alignment = acAlignmentMiddleLeft 'middle-left(ML)
Case "MC"
textObj.Alignment = acAlignmentMiddleCenter 'middle-center(MC)
Case "MR"
textObj.Alignment = acAlignmentMiddleRight 'middle-right(MR)
Case "C"
textObj.Alignment = acAlignmentCenter 'standard-center(C)
Case "R"
textObj.Alignment = acAlignmentRight 'standard-right(R)
Case "BL"
textObj.Alignment = acAlignmentBottomLeft 'bottom-left(BL)
Case "BC"
textObj.Alignment = acAlignmentBottomCenter 'bottom-center(BC)
Case "BR"
textObj.Alignment = acAlignmentBottomRight 'bottom-right(BR)
Case Else
textObj.Alignment = acAlignmentLeft 'Standard left(L)
End Select
'Specify TextAlignmentPoint except when the alignment is to the left of the reference.
If textObj.Alignment <> acAlignmentLeft Then
textObj.TextAlignmentPoint = insertPoint
End If
'Handle Acquisition
Cells(2, 8) = textObj.Handle
'redraw
acadDoc.Regen acActiveViewport
'Show message
MsgBox "Filled in letters!"
End Sub
If the base point cannot be set properly, the code for the base point may not be loaded. This may be due to Excel’s reference settings. In that case, you can assign a base point number.
Function procedure to fill in characters
You can more easily fill in the text by creating the code as a Function procedure. Here we describe how to do this.
First, let’s write the following code in the standard module of EXCEL VBA as it is.
Public acadApp As AcadApplication 'Variable that holds the AutoCAD application document
Public acadDoc As AutoCAD.AcadDocument 'Variable that holds the AutoCAD document object
Function AddTextInAutoCAD(text As String, size As Double, angle As Double, align As String, x1 As Double, y1 As Double, z1 As Double) As String
'Function to add characters to AutoCAD
'Arguments are in order from the beginning.
'Character string to be filled in, character size, character angle, character base point, character X-coordinate, Y-coordinate, Z-coordinate
'The return value is the handle of the drawn character.
Dim textObj As AcadText 'Variable that holds a text object
Dim insertPoint(2) As Double 'Array holding the coordinates of the text
'Set character coordinates
insertPoint(0) = x1
insertPoint(1) = y1
insertPoint(2) = z1
Dim textRotation As Double 'Variable that holds the angle of the character
textRotation = angle * (3.14159265358979 / 180) 'Convert angles to radians
'text generation
Set textobj = acadDoc.modelSpace.AddText(text, insertPoint, size)
'Set text rotation angle
textObj.Rotation = textRotation
'Set character base point
Select Case align
Case "TL"
textObj.Alignment = acAlignmentTopLeft 'top-left(TL)
Case "TC"
textObj.Alignment = acAlignmentTopCenter 'top-center(TC)
Case "TR"
textObj.Alignment = acAlignmentTopRight 'top-right(TR)
Case "ML"
textObj.Alignment = acAlignmentMiddleLeft 'middle-left(ML)
Case "MC"
textObj.Alignment = acAlignmentMiddleCenter 'middle-center(MC)
Case "MR"
textObj.Alignment = acAlignmentMiddleRight 'middle-right(MR)
Case "C"
textObj.Alignment = acAlignmentCenter 'standard-center(C)
Case "R"
textObj.Alignment = acAlignmentRight 'standard-right(R)
Case "BL"
textObj.Alignment = acAlignmentBottomLeft 'bottom-left(BL)
Case "BC"
textObj.Alignment = acAlignmentBottomCenter 'bottom-center(BC)
Case "BR"
textObj.Alignment = acAlignmentBottomRight 'bottom-right(BR)
Case Else
textObj.Alignment = acAlignmentLeft 'Standard left(L)
End Select
'Specify TextAlignmentPoint except when the alignment is to the left of the reference.
If textObj.Alignment <> acAlignmentLeft Then
textObj.TextAlignmentPoint = insertPoint
End If
'Returns the handle of the added line segment
AddTextInAutoCAD = textObj.Handle
End Function
Now, when drawing a line segment, simply call AddTextInAutoCAD(text , size , angle , align , x1 , y1 , z1).
For example, prepare the following sheet, the same as before

Then write a sample of the main procedure. This sample should be executed with AutoCAD open. It draws text in the active drawing.
public Sub main()
On Error GoTo OUT1 'Error Handling: When AutoCAD is not running
Set acadApp =GetObject(,"AutoCAD.Application") 'Retrieve existing AutoCAD applications
On Error GoTo OUT2 'Error Handling: AutoCAD file not opened
Set acadDoc = acadApp.ActiveDocument 'Retrieve the active AutoCAD document
On Error GoTo 0 'Reset error handling
'Draw text in AutoCAD and write the handle to Excel
Cells(2,8) = AddTextInAutoCAD(Cells(2,1), Cells(2,2), Cells(2,3), Cells(2,4), Cells(2,5), Cells(2,6), Cells(2,7))
Exit Sub
OUT1:
MsgBox "Launch AutoCAD"
Exit Sub
OUT2:
MsgBox "Please open the AutoCAD file"
End Sub
Line 11 calls the Function procedure. For the sake of the sample, only one line is described, but if you increase the number of rows in the Excel sheet and use a For statement, you can draw a large number of line segments.
Of course, you can use the following without reading values from cells.
Cells(2,8) = AddTextInAutoCAD("hello", 100, 0, "L", 100, 200, 0)
If you want to draw characters but do not need handles, you can prepare variables such as dummy as shown below.
dummy = AddTextInAutoCAD("hello", 100, 0, "L", 100, 200, 0)
Conclusion
In this article, we showed how to add text to AutoCAD using Excel VBA. We also showed examples of function procedures that can be easily called and used.
By utilizing VBA, you can automate your work in AutoCAD and further improve your design efficiency.
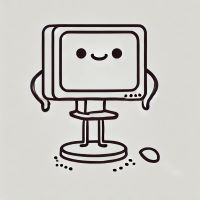
The hardest thing about AutoCAD? Putting text where you want it to be!
コメント