Introduction.
When using FEMAP, you will need to set material properties for many elements. However, doing this manually is time consuming. We will now show you how to use VBA to efficiently create materials in FEMAP.
Material (isotropic) creation code
FEMAP provides an API, which can be called in VBA to perform various operations.
Here is an example of VBA code that creates a new material in FEMAP.
The code must be executed after FEMAP has been started.
Sub create_material
'Generating Femap model objects
Dim f As Object
Set f = GetObject(,"femap.model")
'Creating Material Objects
Dim m As Object
Set m = f.feMatl
m.title = "Steel" 'Enter a title. In this case, the string “Steel” is assigned to the title.
m.Type = 0 'Input for material type, substituting 0 for isotropic material.
m.Ex = 21000 'Substitution of longitudinal modulus of elasticity (Young's modulus)
m.Gx = 80770 'Substitution of transverse modulus of elasticity (shear modulus)
m.Nuxy = 0.3 'Poisson's ratio substitution
m.Alphaxx = 11.7 'Thermal expansion coefficient
m.Kxx = 51.6 'Thermal conductivity
m.Cp = 0.473 'specific heat
m.TensionLimit1 = 235 'Tensile Limiting Stress
m.CompressionLimit1 = 235 'Compression Limiting Stress
m.ShearLimit = 136 'Limiting stress in shear
m.Density = 7.85 'specific gravity
m.Damping = 0.02 'Structural Attenuation Ratio
m.RefTemp = 22 'Reference temperature
m.put(1) 'Material data is input|overwritten to the specified ID. In this case, material data is entered|overwritten at ID=1.
End Sub
- Lines 4-5 create the Femap object; note that executing this code when FEMAP is not running will result in an error.
- Lines 8-9 create the material object of the Entity object.
- Line 11 m.Title = “title” is the assignment of the title, which is a String type.
- Line 12 m.Type = 0 specifies the type of material; assign a long type; 0 is an isotropic material; for other materials, enter a different constant.
- Lines 15-28 You can assign a value for each item. The type of each item is Double. You can omit items that are not set.
- Line 30 m.put(ID) overwrites the number ID data. The ID in parentheses is filled in with the Long type.
Example of linking to an Excel sheet
To create a material with values entered in an Excel sheet
Prepare the following sheet in Excel.
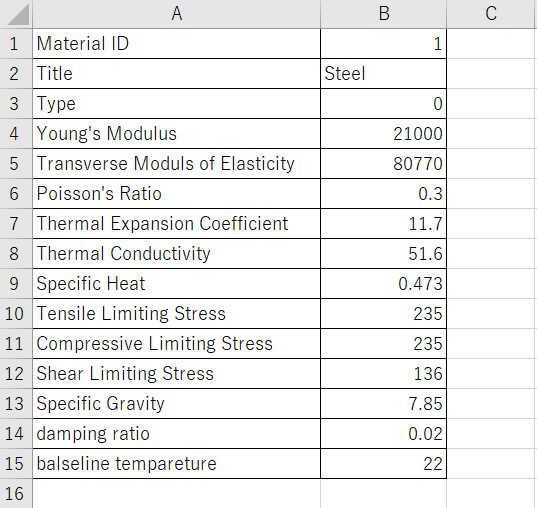
The code to create a material with each value entered on the above sheet is as follows
※Type is the type of material. Here we are dealing with an isotropic material, so enter “0”.
Sub create_material
'Generating Femap model objects
Dim f As Object
Set f = GetObject(,"femap.model")
'Creating Material Objects
Dim m As Object
Set m = f.feMatl
m.title = Cells(2,2) 'Enter a title. In this case, the string “Steel” is assigned to the title.
m.Type = Cells(3,2) 'Input for material type, substituting 0 for isotropic material.
m.Ex = Cells(4,2) 'Substitution of longitudinal modulus of elasticity (Young's modulus)
m.Gx = Cells(5,2) 'Substitution of transverse modulus of elasticity (shear modulus)
m.Nuxy = Cells(6,2) 'Poisson's ratio substitution
m.Alphaxx = Cells(7,2) 'Substitution of thermal expansion coefficient
m.Kxx = Cells(8,2) 'Substitution of thermal conductivity
m.Cp = Cells(9,2) 'Specific Heat Assignment
m.TensionLimit1 = Cells(10,2) 'Substitution of limiting stress in tension
m.CompressionLimit1 = Cells(11,2) 'Substitution of limiting stress for compression
m.ShearLimit = Cells(12,2) 'Substitution of limiting stress for shear
m.Density = Cells(13,2) 'Substitution of specific weightsv
m.Damping = Cells(14,2) 'Substitution of structural damping ratio
m.RefTemp = Cells(15,2) 'Substitution of reference temperature
m.put(Cells(1,2)) 'Input|overwrite material data to the specified ID.
End Sub
If you get an error when retrieving a value from a cell, you can enclose the part of the cell that retrieves the value with Int() if it is a Long type, for example.
Example of exporting material information to ExcelExample of exporting material information to Excel
You can also export FEMAP material information to Excel. In this case, use the .get method.v
First, prepare an Excel sheet as shown below. It is almost the same as the aforementioned example.
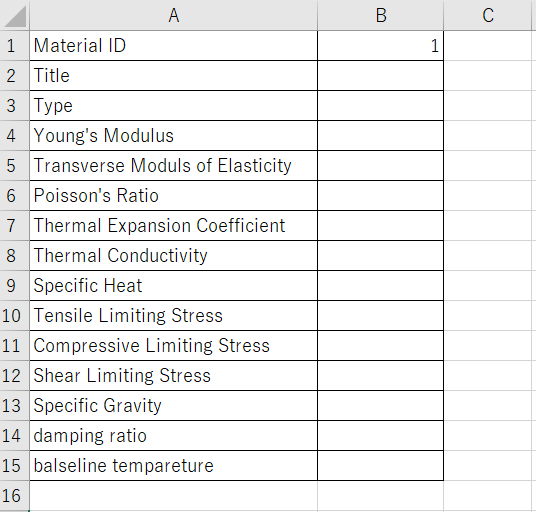
Enter only the ID of the material information you wish to retrieve in the above sheet.
The VBA code is as follows
Sub get_material
'Generating Femap model objects
Dim f As Object
Set f = GetObject(,"femap.model")
'Creating Material Objects
Dim m As Object
Set m = f.feMatl
m.Get(Int(Cells(1,2))) 'Obtains material data for a specified ID
Cells(2,2) = m.title 'Title output
Cells(3,2) = m.Type 'Material type output
Cells(4,2) = m.Ex 'Longitudinal modulus of elasticity (Young's modulus) output
Cells(5,2) = m.Gx 'Lateral modulus of elasticity (shear modulus) output
Cells(6,2) = m.Nuxy 'Poisson's ratio substitution
Cells(7,2) = m.Alphaxx 'Thermal expansion coefficient output
Cells(8,2) = m.Kxx 'Thermal Conductivity Output
Cells(9,2) = m.Cp 'Specific heat output
Cells(10,2) = m.TensionLimit1 'Output of limiting stress in tension
Cells(11,2) = m.CompressionLimit1 'Compression Limiting Stress Output
Cells(12,2) = m.ShearLimit 'Output of limiting stresses in shear
Cells(13,2) = m.Density 'Specific Gravity Output
Cells(14,2) = m.Damping 'Structural Attenuation Ratio Output
Cells(15,2) = m.RefTemp 'Reference temperature output
End Sub
- Line 11 m.get(ID) obtains the data for the number ID. The ID in parentheses is filled in with the Long type. When obtaining cell values, you can reduce the possibility of errors by converting the type, such as Int(Cells(1,2)).
- Running this code will cause the material information to be output to the Excel sheet.
Conclusion
FEMAP’s automatic material creation using EXCEL VBA introduced here is a powerful tool that greatly reduces manual work and improves efficiency when working with complex models. We encourage you to customize it for your own projects.
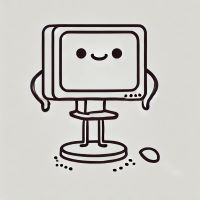
When I analyzed it without setting the material in FEMAP, it looked like a house made of jelly.
コメント