Introduction.
Hello, I’d like to share my experience with you! In this article, I will show you how to create a curve from two points with Femap using Excel VBA, and by using Femap’s API, you can streamline the work you used to do manually!
Code to create a curve (line)
Here is the code to create a curve from two points. Paste the following code into your VBA editor.
As a reminder, the code below requires two points whose point IDs are 1 and 2.
Please see this article for more information on creating points.
Sub CreateCurveLineInFemap()
'Generating Femap model objects
Dim f As Object
Set f = GetObject(,"femap.model")
'Creation of curve object
Dim cu As Object
Set cu = f.feCurve
'Curve (line) setting
cu.Type = 0. 'Type of curve; substitute 0 for line.
cu.stdPoint(0) = 1 'The point ID at which the curve starts, in this case 1.
cu.stdPoint(1) = 2 'The point ID at which the curve starts, in this case 2.
'Creating Curves
cu.put(1) 'Enter the curve ID to be registered. In this case, ID=1.
MsgBox "Curve (line) created."
'Object Release
Set cu = Nothing
Set f = Nothing
End Sub
Code Description
This code creates a curve between point ID=1 and point ID=2 using Femap API.Code
- Lines 4-5 create the Femap object; note that executing this code when FEMAP is not running will result in an error.
- Lines 8-9 create the curve object.
- In line 12, the type of curve is specified by a number. In this case, 0 is assigned since it is a line.
- Lines 13-14 assign the ID of the point that will be the end point of the curve to be created as a Long type.
- Lines 4-5 create the Femap object, and note that executing this code without FEMAP running will result in an error.
- Lines 8-9 create the curve object.
- In line 12, the type of curve is specified by a number. In this case, 0 is assigned since it is a line.
- Lines 13-14 assign the ID of the point that will be the end point of the curve to be created as a Long type.
- The code in line 19 is the key part of creating the curve from the two points.
Example of linking to an Excel sheet
To create a curve with a point ID entered on an Excel sheet
Prepare the following sheet in Excel.
Enter the IDs you wish to designate in A2 to C2.
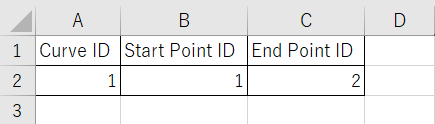
The code to create each value curve entered on the above sheet is as follows
Sub CreateCurveLineInFemap()
'Generating Femap model objects
Dim f As Object
Set f = GetObject(,"femap.model")
'Creation of curve object
Dim cu As Object
Set cu = f.feCurve
'Curve (line) setting
cu.Type = 0. 'Type of curve; substitute 0 for line.
cu.stdPoint(0) = Cells(2, 2) 'Point ID at which the curve begins.
cu.stdPoint(1) = Cells(2, 3) 'Point ID where the curve ends.
'Creating Curves
cu.put(Cells(2, 1)) 'Enter the Curve ID to be registered.
MsgBox "Curve (line) created."
'Object Release
Set cu = Nothing
Set f = Nothing
End Sub
If you get an error when retrieving a value from a cell, you can specify the type of the part that retrieves the value in the cell, such as CLng(Cells(2,1)) for Long type.
To output curve information to Excel
Prepare the following sheet in Excel.
Enter the curve ID in A2 from which you want to extract data.
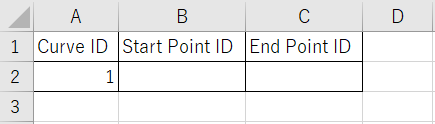
The code to obtain the starting and ending point IDs of the line curve IDs entered on the above sheet is as follows.
Sub GetCurveLineInFemap()
'Generating Femap model objects
Dim f As Object
Set f = GetObject(,"femap.model")
'Creation of curve object
Dim cu As Object
Set cu = f.feCurve
'Obtaining curves (lines)
cu.Get(Cells(2, 1)) 'Obtain information on the curve ID specified here.
'Output of each value
If cu.Type = 0 Then 'If the curve type is 0 (=line)
if cu.stdPoint(0) <> 0 And cu.stdPoint(1) <> 0 Then 'If a start point and an end point exist
Cells(2, 2) = cu.stdPoint(0) 'Point ID at which the curve begins.
Cells(2, 3) = cu.stdPoint(1) 'Point ID where the curve ends.
MsgBox "Obtained curve information for the specified ID."
Else
MsgBox "The curve with the specified ID does not exist."
End If
Else
MsgBox "The curve with the specified ID is not a line."
End If
'Object Release
Set cu = Nothing
Set f = Nothing
End Sub
- Line 12 cu.get(ID) gets the data for the number ID. The ID in parentheses is filled in with the Long type. When retrieving cell values, you can reduce the possibility of errors by converting the type, such as CLong(Cells(1,2)).
- Executing this code will cause the curve information to be output to the Excel sheet.
- The If statement in line 15 only handles the case where the curve is a line.
- The If statement in line 16 determines if the curve exists. If the curve does not exist, the starting and ending point IDs are set to 0.
Conclusion
How was it? In this article, we introduced how to create a Femap curve and how to retrieve information using Excel VBA. By combining the usual Femap operation with Excel, you can automate a time-consuming manual operation or process multiple operations at once, which will greatly improve your work efficiency. Please try it.
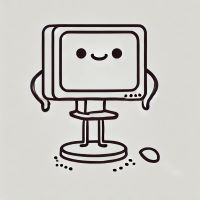
Why are Femap’s lines so close?
Because we all value the “connection points”.
コメント