Introduction.
In this article, we will show you how to draw a circle in AutoCAD using Excel VBA, which allows you to manipulate AutoCAD from Excel to streamline your drawing process.
Preparation of VBA in EXCEL
In order to manipulate AutoCAD with Excel VBA, you need to set references to AutoCAD’s object libraries.
- From the VBA editor menu, select “Tools” > “Reference Settings”.
- Select “AutoCAD Type Library” or “AutoCAD Object Library” from the list, check the box and click “OK”.
(*The name may change slightly depending on the version. AutoCAD 2021 Type Library” etc.)
Sample code to draw circle(s)
Let me show you a sample code that draws a circle using the values entered in Excel.
First, prepare an Excel sheet like the one below.
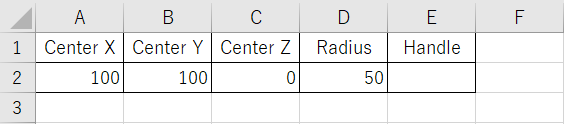
The sequence of cells A2 to D2 are the input cells.
E2 is a cell to get the handle value of the drawn circle. No input is required.
The handle values are obtained because they will be useful later in the automation of AutoCAD, but there is no need to be concerned about them at this stage.
The VBA code is as follows.
Sub DrawCircleInAutoCAD()
'Declare an AutoCAD application object
Dim acadApp As Object
'Declare an AutoCAD document object
Dim acadDoc As Object
'Declare a circle object
Dim circleObj As Object
'If AutoCAD is not running, start it; if it is already open, retrieve it.
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("AutoCAD.Application")
End If
On Error GoTo 0
'Show AutoCAD
acadApp.Visible = True
'If the AutoCAD document is not open, create a new one; if it is open, retrieve it.
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'Declaration of a variable to put the center point of a circle
Dim centerPoint(0 To 2) As Double
'Set the center point
centerPoint(0) = Cells(2,1) 'X-coordinate
centerPoint(1) = Cells(2,2) 'Y-coordinate
centerPoint(2) = Cells(2,3) 'Z-coordinate
'Declaration of a variable to contain the radius
Dim radius As Double
'Radius setting
radius = Cells(2,4) 'radius
'Create circle
Set circleObj = acadDoc.ModelSpace.AddCircle(centerPoint, radius)
'Obtaining a handle
Cells(2,5) = circleObj.Handle
'Display of object range
acadDoc.Application.ZoomExtents
'redraw
acadDoc.Regen acActiveViewport
'Show message
MsgBox ”Circles were drawn!”
End Sub
Circle VBA Function procedure
If you create your code as a Function procedure, you can more easily draw a large number of circles. Here we describe how to do this.
First, let’s write the following code in the standard module of EXCEL VBA as it is.
Public acadApp As AcadApplication 'Variable that holds the AutoCAD application document
Public acadDoc As AutoCAD.AcadDocument 'Variable that holds the AutoCAD document object
Function DrawCircleInAutoCAD(x1 As Double, y1 As Double, z1 As Double, r1 As Double) As String
'Function to draw a circle in AutoCAD
'Arguments are in order from the beginning.
'X coordinate of center, Y coordinate of center, Z coordinate of center, size of radius
'The return value is the handle of the drawn circle.
Dim circleObj As AcadCircle 'Variable that holds a circle object
Dim centerPoint(2) As Double 'Array holding the coordinates of the center
Dim radius As Double 'Variable that holds the radius
'Set center coordinates
centerPoint(0) = x1
centerPoint(1) = y1
centerPoint(2) = z1
'Set radius
radius = r1
'Adding circles to AutoCAD model space
Set circleObj = acadDoc.ModelSpace.AddCircle(centerPoint, radius)
'Returns the handle of the added circle
DrawCircleInAutoCAD = circleObj.Handle
End Function
Now it is easy to use DrawCircleInAutoCAD(x1,y1,z1,r1) to draw a circle.
For example, prepare the following sheet.
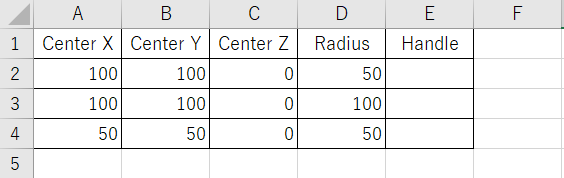
Then write a sample of the main procedure. This sample should be executed with AutoCAD open. Draw a circle in the active drawing.
public Sub main()
On Error GoTo OUT1 'Error Handling: When AutoCAD is not running
Set acadApp =GetObject(,"AutoCAD.Application") 'Retrieve existing AutoCAD applications
On Error GoTo OUT2 'Error Handling: AutoCAD file not opened
Set acadDoc = acadApp.ActiveDocument 'Retrieve the active AutoCAD document
On Error GoTo 0 'Reset error handling
'Draw a circle in AutoCAD and write its handle to Excel
Cells(2,5) = DrawCircleInAutoCAD(Cells(2,1),Cells(2,2),Cells(2,3),Cells(2,4))
Cells(3,5) = DrawCircleInAutoCAD(Cells(3,1),Cells(3,2),Cells(3,3),Cells(3,4))
Cells(4,5) = DrawCircleInAutoCAD(Cells(4,1),Cells(4,2),Cells(4,3),Cells(4,4))
Exit Sub
OUT1:
MsgBox "Launch AutoCAD"
Exit Sub
OUT2:
MsgBox "Please open the AutoCAD file"
End Sub
Lines 11 to 13 are calling the Function procedure. For the sake of the sample, I am describing each one individually, but you can draw a large number of circles by using a For statement, etc.
Of course, you can use the following without reading values from cells.
Cells(2,5) = DrawCircleInAutoCAD(0,0,0,10) 'Draw a circle of radius 10 at center point (0,0,0)
If you want to draw a circle but do not need a handle, you can prepare a variable such as dummy as shown below.
dummy = DrawCircleInAutoCAD(0,0,0,10) 'Draw a circle of radius 10 at center point (0,0,0)
Conclusion
In this article, we explained the basic method of drawing circles in AutoCAD using Excel VBA. By applying this method, you can draw figures in AutoCAD based on data entered in Excel and automate drafting operations. Please start with simple shapes and gradually try more complex operations.
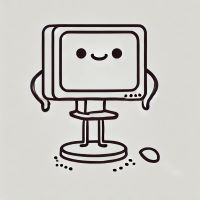
Using Excel VBA to draw circles in AutoCAD? That’s a lot less mistakes and a lot less corners!
コメント