Introduction
In this article, I will show you how to draw arc(s) in AutoCAD using Excel VBA, which may sound a bit difficult when you hear the word VBA, but if you follow these steps, you will be able to do it. In the second half of the presentation, we will also introduce the Fuction procedure, which you will be able to use easily.
Preparation of VBA in EXCEL
In order to manipulate AutoCAD with Excel VBA, the AutoCAD object library must be set as a reference.
- Select “Tools” -> “Reference Settings” from the VBA Editor menu.
- Select “AutoCAD Type Library” or “AutoCAD Object Libraly” from the list, check the box and click “OK”.
(*The name may change slightly depending on the version. AutoCAD 2021 Type Library” etc.)
Sample code to draw arc(s)
Let me show you a sample code that draws an arc using the values entered into Excel.
First, prepare an Excel sheet like the one below.

The sequence of cells A2 to F2 are the input cells.
G2 is a cell for obtaining the handle value of the drawn arc. No input is necessary when drawing.
The handle values are obtained because they will be useful later in the automation of AutoCAD, but you do not need to worry about them if you are just drawing.
The VBA code is as follows.
Sub DrawCircleInAutoCAD()
'Declare an AutoCAD application object
Dim acadApp As Object
'Declare an AutoCAD document object
Dim acadDoc As Object
'Declare arc object
Dim arcObj As Object
'If AutoCAD is not running, start it; if it is already open, retrieve it.
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("AutoCAD.Application")
End If
On Error GoTo 0
'Show AutoCAD
acadApp.Visible = True
'If the AutoCAD document is not open, create a new one; if it is open, retrieve it.
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'Declaration of a variable to put the center point of a circle
Dim centerPoint(0 To 2) As Double
'Set the center point
centerPoint(0) = Cells(2,1) 'X-coordinate
centerPoint(1) = Cells(2,2) 'Y-coordinate
centerPoint(2) = Cells(2,3) 'Z-coordinate
'Declaration of a variable to contain the radius
Dim radius As Double
'Radius setting
radius = Cells(2,4) 'radus
'Set the beginning and end angles of the arc.
startAngle = cells(2,5) * (3.14159265358979 / 180) 'Convert angles to radians
endAngle = cells(2,6) * (3.14159265358979 / 180) 'Convert angles to radians
'Create arc
Set arcObj = acadDoc.ModelSpace.AddArc(centerPoint, radius, startAngle, endAngle)
'Obtaining a handle
Cells(2,7) = arcObj.Handle
'Display of object range
acadDoc.Application.ZoomExtents
'redraw
acadDoc.Regen acActiveViewport
'Show message
MsgBox ”An arc was drawn!”
End Sub
VBA Function procedure to draw an arc
If you create your code as a Function procedure, you can more easily draw a large number of arcs. Here we describe how to do this.
First, let’s write the following code in the standard module of EXCEL VBA as it is.
Public acadApp As AcadApplication 'Variable that holds the AutoCAD application document
Public acadDoc As AutoCAD.AcadDocument 'Variable that holds the AutoCAD document
Function DrawArcInAutoCAD(x1 As Double, y1 As Double, z1 As Double, r1 As Double, a1 As Double, a2 As Double) As String
'Function to draw a circular arc in AutoCAD
'Arguments are in order from the beginning.
'X coordinate of the center, Y coordinate of the center, Z coordinate of the center, size of the radius, beginning angle of the arc, end angle of the arc
'The return value is the handle of the drawn arc.
Dim arcObj As AcadArc 'Variable that holds the arc object
Dim centerPoint(2) As Double 'Array holding the coordinates of the center
Dim radius As Double 'Variable that holds the radius
Dim startAngle As Double 'Bigining angle of arc
Dim endAngle As Double 'End angle of arc
'Set center coordinates
centerPoint(0) = x1
centerPoint(1) = y1
centerPoint(2) = z1
'Set radius
radius = r1
'Set the angle of the arc
startAngle = a1 * (3.14159265358979 / 180) 'Convert angles to radians
endAngle = a2 * (3.14159265358979 / 180) 'Convert angles to radians
'Adding arcs to AutoCAD model space
Set arcObj = acadDoc.ModelSpace.AddArc(centerPoint, radius, startAngle, endAngle)
'Returns the handle of the added arc
DrawArcInAutoCAD = arcObj.Handle
End Function
Now it is easy to use DrawArcInAutoCAD(x1,y1,z1,r1,a1,a2) to draw a circular arc.
For example, prepare the following sheet.
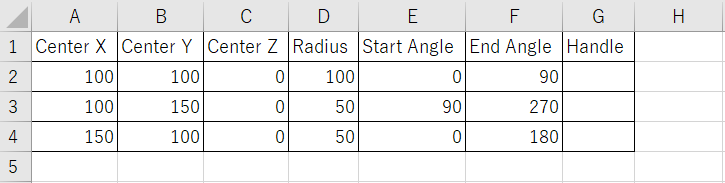
Then write a sample of the main procedure.
This sample should be run with AutoCAD open. Draw an arc in the active drawing.
public Sub main()
On Error GoTo OUT1 'Error Handling: When AutoCAD is not running
Set acadApp =GetObject(,"AutoCAD.Application") 'Retrieve existing AutoCAD applications
On Error GoTo OUT2 'Error Handling: AutoCAD file not opened
Set acadDoc = acadApp.ActiveDocument 'Retrieve the active AutoCAD document
On Error GoTo 0 'Reset error handling
'Draw an arc in AutoCAD and write the handle to Excel
Cells(2,7) = DrawArcInAutoCAD(Cells(2,1), Cells(2,2), Cells(2,3), Cells(2,4), Cells(2,5), Cells(2,6))
Cells(3,7) = DrawArcInAutoCAD(Cells(3,1), Cells(3,2), Cells(3,3), Cells(3,4), Cells(3,5), Cells(3,6))
Cells(4,7) = DrawArcInAutoCAD(Cells(4,1), Cells(4,2), Cells(4,3), Cells(4,4), Cells(4,5), Cells(4,6))
Exit Sub
OUT1:
MsgBox "Launch AutoCAD"
Exit Sub
OUT2:
MsgBox "Please open the AutoCAD file"
End Sub
Lines 11 to 13 are calling the Function procedure. For the sake of the sample, I have described them one by one, but you can draw a large number of arcs if you use a For statement.
Of course, you can use the following without reading values from cells.
Cells(2,7) = DrawArcInAutoCAD(0, 0, 0, 10, 0, 90) 'Draw an arc with center point (0,0,0) and radius 10, from 0 to 90 degrees
If you want to draw a circular arc but do not need handles, you can prepare variables such as dummy as shown below.
dummy = DrawArcInAutoCAD(0, 0, 0, 10, 0, 90) 'Draw an arc with center point (0,0,0) and radius 10, from 0 to 90 degrees
Conclusion
In this article, we explained the basic method of drawing arcs in AutoCAD using Excel VBA. By applying this method, you can draw figures in AutoCAD based on data entered in Excel and automate drafting operations. Please start with simple shapes and gradually try more complex operations.
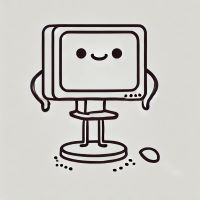
Drawing arcs in VBA? Well, Excel is always surrounded by square cells, so you long for the beauty of curves.
コメント