Introduction
In this article we will explain how to automatically add points to Femap using Excel VBA, a fun way to use coordinate data written in Excel to create points in a snap without relying on the Femap interface. This is a must-have technique for engineers to reduce manual work and create points efficiently.
Point creation code
Now it’s time to get down to business. Let’s write the following code in the VBA module.
Sub CreteFemapPoint()
Dim feApp As Object
Dim pt As Object
'Get Femap application
Set feApp = GetObject(, "femap.model")
Set pt = feApp.fePoint
'Point generation
pt.X = 15 'Input X-coordinates
pt.Y = 25 'Input Y-coordinates
pt.Z = 35 'Input Z-coordinates
pt.put(1) 'Generate (overwrite) points to the specified ID
'View Results
MsgBox "Point created."
'object release
Set pt = Nothing
Set feApp = Nothing
End Sub
Explanation of Codes
This code uses FEMAP’s API to generate points.
- Lines 2 to 7 create a Femap object.
Note that executing this code when Femap is not running will result in an error. - In lines 10 to 12, the coordinates of the point to be created are input. The coordinates are entered as Double type.
- In line 13, pt.put(ID) overwrites the data with the number ID. The ID in parentheses is entered with the Long type.
Example of linking to an Excel sheet
To create points with values entered in an Excel sheet
Prepare the following sheet in Excel.
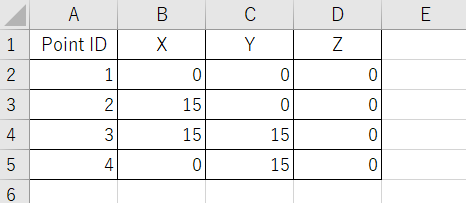
The code to create a point with each value entered on the above sheet is as follows.
Sub CrateFemapPoint()
Dim i As Integer
'Generate Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Generating Point Objects
Dim pt As Object
Set pt = f.fePoint
For i = 2 To 5
pt.X = Cells(i, 2)
pt.Y = Cells(i, 3)
pt.Z = Cells(i, 4)
pt.Put(Cells(i, 1)) 'Overwrites or generates points to the specified ID.
Next i
MsgBox "Points have been created!"
End Sub
If an error occurs when retrieving a value from a cell, it may be better to clarify the type of value to be retrieved by enclosing the part of the cell to be retrieved with CLng() for Long type, CDbl() for Double type, etc.
Example of exporting point coordinates to Excel
You can also export the coordinates of the points in the Femap to Excel. In this case, use the .get method.
First, prepare the following Excel sheet. It is almost the same as the previous example, but the coordinates are empty.
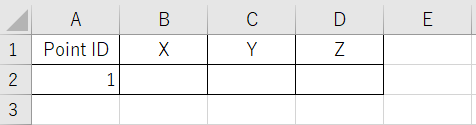
Enter only the ID of the point you wish to retrieve on the above sheet.
The VBA code would look like this
Sub getPoint()
'Generate Femap model object
Dim f As Object
Set f = GetObject(,"femap.model")
'Generating Point Objects
Dim pt As Object
Set pt = f.fePoint
pt.Get(Int(Cells(2, 1))) 'Obtains point data for a specified ID
Cells(2, 2) = pt.X 'Obtaining X-coordinates
Cells(2, 3) = pt.Y 'Obtaining Y-coordinates
Cells(2, 4) = pt.Z 'Obtaining Z-coordinates
End Sub
The data of the number ID is obtained by pt.get(ID) in line 10. The ID in parentheses is filled in with the Lomg type.
By executing this code, you can have the point information output to the excel sheet.
conclusion
This new point creation code makes the automatic operation from Excel to FEMAP even smoother. This saves time and effort and improves accuracy.
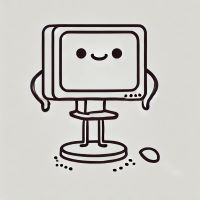
When I added too many points to FEMAP, they got mad at me for “wasting too many points”.
コメント