Introduction.
AutoCAD is a powerful CAD tool, and automation with Excel VBA can make your design work more efficient. This article explains how to draw AutoCAD line segments using Excel VBA.
Preparation of VBA in EXCEL
In order to manipulate AutoCAD with Excel VBA, the AutoCAD object library must be set as a reference.
- Select “Tools” -> “Reference Settings” from the VBA Editor menu.
- Select “AutoCAD Type Library” or “AutoCAD Object Libraly” from the list, check the box and click “OK”.
(*The name may change slightly depending on the version. AutoCAD 2021 Type Library” etc.)
Sample code to draw a line
Let me show you a sample code to draw a line segment using the values entered in Excel.
First, prepare an Excel sheet like the one below.

The sequence of cells A2 to F2 are the input cells.
G2 is a cell to get the handle value of the drawn line segment. No input is required.
The handle values will be important later in the process of automating AutoCAD, so we are trying to obtain them, but at this stage we do not need to worry about them.
The VBA code is as follows.
Sub DrawLineInAutoCAD()
'Declare an AutoCAD application object
Dim acadApp As Object
'Declare an AutoCAD document object
Dim acadDoc As Object
'Declare a line object
Dim lineObj As Object
'If AutoCAD is not running, start it; if it is already open, retrieve it.
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("AutoCAD.Application")
End If
On Error GoTo 0
'Display AutoCAD
acadApp.Visible = True
'If the AutoCAD document is not open, create a new one; if it is open, retrieve it.
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'Declaration of a variable to contain the starting and ending points
Dim startPoint(0 To 2) As Double
Dim endPoint(0 To 2) As Double
'Set the starting point
startPoint(0) = Cells(2,1) 'X-coordinate
startPoint(1) = Cells(2,2) 'Y-coordinate
startPoint(2) = Cells(2,3) 'Z-coordinate
'Set the ending point
endPoint(0) = Cells(2,4) 'X-coordinate
endPoint(1) = Cells(2,5) 'Y-coordinate
endPoint(2) = Cells(2,6) 'Z-coordinate
'Create a line
Set lineObj = acadDoc.ModelSpace.AddLine(startPoint, endPoint)
'Obtaining a handle
Cells(2,7) = lineObj.Handle
'Display of object range
acadDoc.Application.ZoomExtents
'redraw
acadDoc.Regen acActiveViewport
'Show message
MsgBox ”Line(s) drawn!”
End Sub
VBA Function procedure for drawing a line
If you create your code as a Function procedure, you can more easily draw large numbers of line segments. Here we describe how to do this.
First, let’s write the following code in the standard module of EXCEL VBA as it is.
Public acadApp As AcadApplication 'Variable that holds the AutoCAD application document
Public acadDoc As AutoCAD.AcadDocument 'Variable that holds the AutoCAD document object
Function DrawLineInAutoCAD(x1 As Double, y1 As Double, z1 As Double, x2 As Double, y2 As Double, z2 As Double) As String
'Functions for drawing lines in AutoCAD
'Arguments are in order from the beginning
'Start Point X, Start Point Y, Start Point Z, End Point X, End Point Y, End Point Z
'Returns the handle of the drawn line
Dim lineObj As AcadLine 'Variable that stores a line object
Dim startPoint(2) As Double, endPoint(2) As Double 'Valiable that stores start points and end points
'Set the start points
startPoint(0) = x1
startPoint(1) = y1
startPoint(2) = z1
'Set the end points
endPoint(0) = x2
endPoint(1) = y2
endPoint(2) = z2
'Add a line to AutoCAD's model space
Set lineObj = acadDoc.ModelSpace.AddLine(startPoint, endPoint)
'Return the handle of the added line
DrawLineInAutoCAD = lineObj.Handle
End Function
Now drawing a line segment is as easy as calling DrawLineInAutoCAD(x1,y1,z1,x2,y2,z2).
For example, prepare the following sheet.
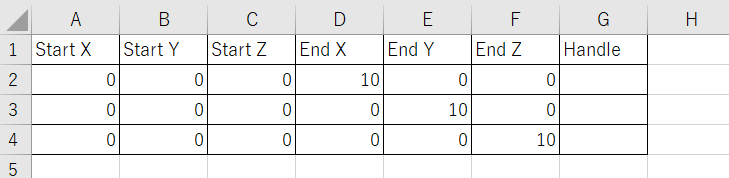
Then write a sample of the main procedure. This sample should be executed with AutoCAD open. It draws a line segment in the active drawing.
public Sub main()
On Error GoTo OUT1 'Error Handling: When AutoCAD is not running
Set acadApp =GetObject(,"AutoCAD.Application") 'Retrieve existing AutoCAD applications
On Error GoTo OUT2 'Error Handling: AutoCAD file not opened
Set acadDoc = acadApp.ActiveDocument 'Retrieve the active AutoCAD document
On Error GoTo 0 'Reset error handling
'Draw a line segment in AutoCAD and write the handle to Excel
Cells(2,7) = DrawLineInAutoCAD(Cells(2,1),Cells(2,2),Cells(2,3),Cells(2,4),Cells(2,5),Cells(2,6))
Cells(3,7) = DrawLineInAutoCAD(Cells(3,1),Cells(3,2),Cells(3,3),Cells(3,4),Cells(3,5),Cells(3,6))
Cells(4,7) = DrawLineInAutoCAD(Cells(4,1),Cells(4,2),Cells(4,3),Cells(4,4),Cells(4,5),Cells(4,6))
Exit Sub
OUT1:
MsgBox "Activate AutoCAD"
Exit Sub
OUT2:
MsgBox "Open an AutoCAD file"
End Sub
Lines 11 to 13 are calling the Function procedure. For the sake of the sample, I am describing each line one by one, but if you use a For statement, you can draw a large number of line segments.
Of course, you can use the following without reading values from cells.
Cells(2,7) = DrawLineInAutoCAD(1,1,0,10,10,0) 'Draw line from Start point(1,1,0) to End point(10,10,0)
If you want to draw a line segment but do not need a handle, you can prepare a variable such as dummy as shown below.
dummy = DrawLineInAutoCAD(1,1,0,10,10,0) 'Draw line from Start point(1,1,0) to End point(10,10,0)
Conclusion
In this article, we showed you how to draw line segments in AutoCAD using Excel VBA. We hope you now understand the basic AutoCAD connections and the flow of code to draw line segments.
By utilizing VBA, you can automate your AutoCAD work and further improve your design efficiency.
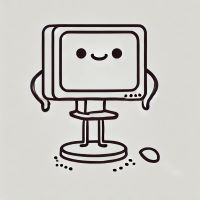
I’ve drawn a lot of line segments lately, and I’m straight-up tired!
See you then.
コメント