はじめに
AutoCADとExcel VBAの連携は、業務効率を大幅に向上させる方法の一つです。この記事では、Excel VBAを使ってAutoCADにテキストを挿入する方法を紹介します。
EXCELでのVBAの準備
Excel VBAでAutoCADを操作するために、AutoCADのオブジェクトライブラリを参照設定する必要があります。
- VBAエディタのメニューから「ツール」→「参照設定」を選択します。
- リストから「AutoCAD Type Library」または「AutoCAD Object Libraly」を選択し、チェックを入れて「OK」をクリックします。
(※Verにより多少名称が変わるかもしれません。「AutoCAD 2021 Type Library」など。)
文字列(テキスト)を挿入するサンプルコード
それでは、エクセルに入力した値を使って文字を挿入するサンプルコードを紹介します。
まずは下のようなエクセルシートを準備します。

A2~G2までが入力セルです。H2は挿入した文字列のハンドルを取得するためのセルです。作図時は入力不要です。テキスト基準位置は次のように設定しました。
基点位置 | Excel入力文字 | VBAコード | 基点番号 |
---|---|---|---|
左上 | TL | acAlignmentTopLeft | 6 |
上中心 | TC | acAlignmentTopCenter | 7 |
右上 | TR | acAlignmentTopRight | 8 |
左中央 | ML | acAlignmentMiddleLeft | 9 |
中央中心 | MC | acAlignmentMiddleCenter | 10 |
右中央 | MR | acAlignmentMiddleRight | 11 |
基準左 | L | acAlignmentLeft | 0 |
基準中央 | C | acAlignmentCenter | 1 |
基準右 | R | acAlignmentRight | 2 |
左下揃え | BL | acAlignmentBottomLeft | 12 |
中央下揃え | BC | acAlignmentBottomCenter | 13 |
右下揃え | BR | acAlignmentBottomRight | 14 |
VBAコードはつぎのようになります。
Sub AddTextInAutoCAD()
Dim acadApp As Object 'AutoCADアプリケーションオブジェクトを宣言
Dim acadDoc As Object 'AutoCADドキュメントオブジェクトを宣言
Dim textObj As Object '文字オブジェクトを宣言
Dim insertPoint(0 To 2) As Double '文字の挿入基点
Dim text As String '記入する文字列の内容
Dim textHeight As Double '文字の大きさ
Dim textRotation As Double '文字の角度
Dim textAlignment As String '文字基点
'AutoCADを起動または取得
On Error Resume Next
Set acadApp = GetObject(,"AutoCAD.Application")
If acadApp Is Nothing Then
Set acadApp = CreateObject("AutoCAD.Application")
End If
On Error GoTo 0
'AutoCADを表示
acadApp.Visible = True
'AutoCADドキュメントを新規作成、または取得する。
On Error Resume Next
Set acadDoc = acadApp.ActiveDocument
If acadDoc Is Nothing Then
Set acadDoc = acadApp.Documents.Add
End If
On Error GoTo 0
'文字の挿入位置、内容、サイズ、角度の設定
text = Cells(2,1) '文字内容
textHeight = Cells(2,2) '文字の大きさ
textRotation = Cells(2,3) * (3.14159265358979 / 180) '文字角度。ラジアンに換算。
textAlignment = Cells(2,4) '文字基点
insertPoint(0) = Cells(2,5) 'X座標
insertPoint(1) = Cells(2,6) 'Y座標
insertPoint(2) = Cells(2,7) 'Z座標
'文字の生成
Set textObj = acadDoc.modelSpace.AddText(text, insertPoint, textHeight)
'文字の回転角度を設定
textObj.Rotation = textRotation
'文字の基点を設定
Select Case textAlignment
Case "TL"
textObj.Alignment = acAlignmentTopLeft '左上(TL)
Case "TC"
textObj.Alignment = acAlignmentTopCenter '上中心(TC)
Case "TR"
textObj.Alignment = acAlignmentTopRight '右上(TR)
Case "ML"
textObj.Alignment = acAlignmentMiddleLeft '中央左(ML)
Case "MC"
textObj.Alignment = acAlignmentMiddleCenter '中央中心(MC)
Case "MR"
textObj.Alignment = acAlignmentMiddleRight '右中央(MR)
Case "C"
textObj.Alignment = acAlignmentCenter '基準中心(C)
Case "R"
textObj.Alignment = acAlignmentRight '基準右(R)
Case "BL"
textObj.Alignment = acAlignmentBottomLeft '左下(BL)
Case "BC"
textObj.Alignment = acAlignmentBottomCenter '下中心(BC)
Case "BR"
textObj.Alignment = acAlignmentBottomRight '右下(BR)
Case Else
textObj.Alignment = acAlignmentLeft '基準左(L)
End Select
'位置合わせが基準左の時以外は、TextAlignmentPointを指定する
If textObj.Alignment <> acAlignmentLeft Then
textObj.TextAlignmentPoint = insertPoint
End If
'ハンドル取得
Cells(2, 8) = textObj.Handle
'再作図
acadDoc.Regen acActiveViewport
'メッセージを表示
MsgBox "文字を記入しました!"
End Sub
基点がうまく設定できないときは、基点のコードが読み込まれてない場合があります。エクセルの参照設定が原因かもしれません。そのときは、基点番号を代入するとよいです。
文字を記入するFunctionプロシージャ
コードをFunctionプロシージャとして作成すれば、もっと容易にテキストを記入することが出来るようになります。ここでは、その方法を記述します。
まず、EXCEL VBAの標準モジュールの中に下記コードをこのまま記述しましょう。
Public acadApp As AcadApplication 'AutoCADのアプリケーションドキュメントを保持する変数
Public acadDoc As AutoCAD.AcadDocument 'AutoCADのドキュメントオブジェクトを保持する変数
Function AddTextInAutoCAD(text As String, size As Double, angle As Double, align As String, x1 As Double, y1 As Double, z1 As Double) As String
'AutoCADに文字を追加する関数
'引数は最初から順に
'記入する文字列、文字の大きさ、文字の角度、文字の基点、文字のX座標、Y座標、Z座標
'戻り値は描画した文字のハンドル
Dim textObj As AcadText 'テキストオブジェクトを保持する変数
Dim insertPoint(2) As Double 'テキストの座標を保持する配列
'文字の座標を設定
insertPoint(0) = x1
insertPoint(1) = y1
insertPoint(2) = z1
Dim textRotation As Double '文字の角度を保持する変数
textRotation = angle * (3.14159265358979 / 180) '角度をラジアンに換算
'文字の生成
Set textobj = acadDoc.modelSpace.AddText(text, insertPoint, size)
'文字の回転角度を設定
textObj.Rotation = textRotation
'文字の基点を設定
Select Case align
Case "TL"
textObj.Alignment = acAlignmentTopLeft '左上(TL)
Case "TC"
textObj.Alignment = acAlignmentTopCenter '上中心(TC)
Case "TR"
textObj.Alignment = acAlignmentTopRight '右上(TR)
Case "ML"
textObj.Alignment = acAlignmentMiddleLeft '中央左(ML)
Case "MC"
textObj.Alignment = acAlignmentMiddleCenter '中央中心(MC)
Case "MR"
textObj.Alignment = acAlignmentMiddleRight '右中央(MR)
Case "C"
textObj.Alignment = acAlignmentCenter '基準中心(C)
Case "R"
textObj.Alignment = acAlignmentRight '基準右(R)
Case "BL"
textObj.Alignment = acAlignmentBottomLeft '左下(BL)
Case "BC"
textObj.Alignment = acAlignmentBottomCenter '下中心(BC)
Case "BR"
textObj.Alignment = acAlignmentBottomRight '右下(BR)
Case Else
textObj.Alignment = acAlignmentLeft '基準左(L)
End Select
'位置合わせが基準左の時以外は、TextAlignmentPointを指定する
If textObj.Alignment <> acAlignmentLeft Then
textObj.TextAlignmentPoint = insertPoint
End If
'追加した線分のハンドルを返す
AddTextInAutoCAD = textObj.Handle
End Function
これで線分を描くときはAddTextInAutoCAD(text , size , angle , align , x1 , y1 , z1)を呼び出すだけで簡単に使えます。
例えば、先ほどと同じ、次のようなシートを準備します。

そしてメインのプロシージャのサンプルを記述します。このサンプルはAutoCADを開いた状態で実行してください。アクティブになっている図面に文字を描きます。
public Sub main()
On Error GoTo OUT1 'エラーハンドリング:AutoCADが起動していないとき
Set acadApp =GetObject(,"AutoCAD.Application") '既存のAutoCADアプリケーションの取得
On Error GoTo OUT2 'エラーハンドリング:AutoCADのファイルが開かれてない場合
Set acadDoc = acadApp.ActiveDocument 'アクティブなAutoCADドキュメントを取得
On Error GoTo 0 'エラーハンドリングをリセット
'AutoCADに文字を描画し、そのハンドルをExcelに書き込む
Cells(2,8) = AddTextInAutoCAD(Cells(2,1), Cells(2,2), Cells(2,3), Cells(2,4), Cells(2,5), Cells(2,6), Cells(2,7))
Exit Sub
OUT1:
MsgBox "AutoCADを起動してください"
Exit Sub
OUT2:
MsgBox "AutoCADのファイルを開いてください"
End Sub
11行目がFunctionプロシージャを呼び出しているところです。サンプルのためひとつだけ記述してますが、エクセルシートの行を増やし、For文など使用すれば大量に線分を描くことが出来るでしょう。
もちろん、セルから値を読み込まなくても下記のように使用できます。
Cells(2,8) = AddTextInAutoCAD("てすと", 100, 0, "L", 100, 200, 0)
文字を描きたいけれどハンドルは不要という場合は、下記のように適当にdummyなどの変数を用意して対応すればよいでしょう。
dummy = AddTextInAutoCAD("てすと", 100, 0, "L", 100, 200, 0)
おわりに
今回の記事では、Excel VBAを使用してAutoCADに文字を追加する方法を紹介しました。また、簡単に呼び出して使用できるファンクションプロシージャの例も紹介しました。
VBAを活用することで、AutoCADでの作業を自動化し、設計の効率をさらに高めることが出来ます。
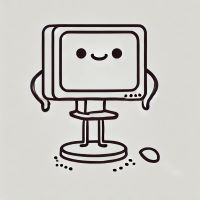
AutoCADで一番難しいこと?テキストを思った場所に置くこと!
コメント